Actions vs Repositories in Laravel
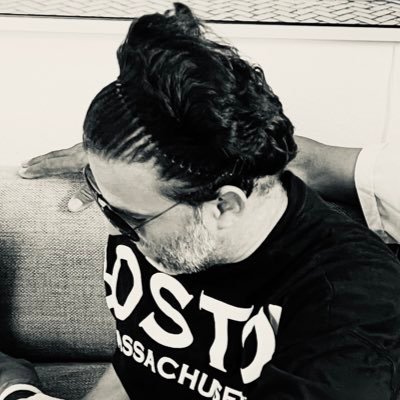
In Laravel, there are different approaches to organizing code when interacting with models and business logic. Two common patterns are Actions and Repositories. Here’s a breakdown of each and how they compare:
Actions (Action Classes) in Laravel
-
Purpose: Actions encapsulate a single task or business logic in a reusable class. Instead of putting logic in controllers or models, actions focus on keeping the logic separated and reusable.
-
Use Case: Useful for scenarios where you have a single responsibility, such as user registration, order processing, or notifications.
-
Organization: Actions are usually located in a directory like
app/Actions
orapp/Services
. Each action class has a method likeexecute
orhandle
that performs the task. -
Example:
class CreateUserAction { public function execute(array $data) { return User::create($data); } }
-
Advantages:
- Clear single responsibility for each action.
- Makes your code more readable and easier to test.
- Reusability across different parts of the application.
-
Disadvantages:
- Can lead to many small classes if used excessively.
- Not always necessary for simple operations.
Repositories in Laravel
-
Purpose: Repositories abstract the database logic away from the controller or business logic. Instead of querying models directly in controllers, the repository pattern creates an intermediary layer.
-
Use Case: Useful for managing complex queries and handling data access in a clean, organized way. Helps in keeping controllers thin by removing query logic.
-
Organization: Repositories are usually located in
app/Repositories
, with interfaces and implementations separated. -
Example:
interface UserRepositoryInterface { public function findByEmail($email); } class UserRepository implements UserRepositoryInterface { public function findByEmail($email) { return User::where('email', $email)->first(); } }
-
Advantages:
- Decouples data access logic from the controller.
- Easier to swap out data storage (e.g., replace Eloquent ORM with another data source).
- Helps with unit testing by mocking repositories instead of hitting the database.
-
Disadvantages:
- Can add unnecessary complexity for simple applications.
- Overhead in creating repository interfaces and implementations.
Comparison
Aspect | Actions | Repositories |
Purpose | Encapsulate business logic or a single task. | Encapsulate data access and queries. |
Use Case | When you want to separate business logic. | When you want to organize query logic. |
Complexity | Lower complexity, but can lead to many classes. | Higher complexity with more structure. |
Reusability | Reusable business logic in multiple places. | Reusable query logic and database interactions. |
Testing | Makes testing specific business logic easier. | Easier to mock for unit tests involving data. |
When to Use Each
- Use Actions if your main goal is to separate out business logic and make it reusable, without focusing too much on data abstraction.
- Use Repositories if you are dealing with complex query logic and want to decouple the database layer from the business logic.
Both patterns can be used together if needed, but it’s important to evaluate the complexity of your application and avoid over-engineering.