Laravel 10.19 Released
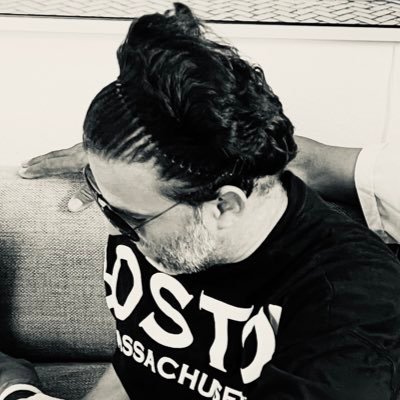
This week, the Laravel team released v10.19 with a collection percentage method, custom event discovery class resolution, dynamic queue listener delay, and more:
Word wrap string method
Josh Bonnick contributed a wordWrap
method to the Laravel String API, which splits strings within a string using a character limit. This method is a wrapper for PHP's wordwrap function:
use Illuminate\Support\Str;
$text = "A very long woooooooooooord."
// Str
Str::wordWrap(string: $text, characters: 8);
/*
A very
long
wooooooo
ooooord.
*/
// Stringable
str($text)->wordWrap(string: $text, characters: 8);
Add percentage method to collections
Wendell Adriel contributed a percentage()
method to collections that allows you to calculate a percentage based on custom logic using a truth
test:
$collection = new $collection([
['name' => 'Taylor', 'foo' => 'foo'],
['name' => 'Nuno', 'foo' => 'bar'],
['name' => 'Dries', 'foo' => 'bar'],
['name' => 'Jess', 'foo' => 'baz'],
]);
$collection->percentage(fn ($value) => $value['foo'] === 'foo'); // 25.00
$collection->percentage(fn ($value) => $value['foo'] === 'bar'); // 50.00
$collection->percentage(fn ($value) => $value['foo'] === 'baz'); // 25.00
$collection->percentage(fn ($value) => $value['foo'] === 'test'); // 0.00
Ability to customize class resolution in Event discovery
@bastien-phi contributed the ability to customize class resolution for Event discovery:
This PR adds the ability to customize the way the translation is done and unlocks event discovery in such DDD structures.
Here's an example of a custom callback in Pull Request #48031's tests, which illustrates how this can be used:
use Illuminate\Foundation\Events\DiscoverEvents;
DiscoverEvents::guessClassNamesUsing(function (SplFileInfo $file, $basePath) {
return Str::of($file->getRealPath())
->after($basePath.DIRECTORY_SEPARATOR)
->before('.php')
->replace(DIRECTORY_SEPARATOR, '\\')
->ucfirst()
->prepend('Illuminate\\')
->toString();
});
Specify listener delay dynamically
@CalebW contributed support for listeners to define a withDelay()
method that can determine queue listener delay:
class Listener implements ShouldQueue
{
public function __invoke(Event $event): void
{
// ...
}
public function withDelay(Event $event): int
{
return $event->fooBar
? <short_delay>
: <long_delay>;
}
}
Add dynamic return types to the rescue helper
Choraimy Kroonstuiver contributed a PHPDoc update to help static analysis tools understand the generic return types of the rescue() helper. There are no code examples to see here, but the updated Docblock looks as follows after this update:
/**
* Catch a potential exception and return a default value.
*
* @template TRescueValue
* @template TRescueFallback
*
* @param callable(): TRescueValue $callback
* @param (callable(\Throwable): TRescueFallback)|TRescueFallback $rescue
* @param bool|callable $report
* @return TRescueValue|TRescueFallback
*/
function rescue(callable $callback, $rescue = null, $report = true)
{
// ...
}
// Examples:
assertType('int|null', rescue(fn () => 123));
assertType('int', rescue(fn () => 123, 345));
assertType('int', rescue(fn () => 123, fn () => 345));
You can learn more about Generics By Examples on the PHPStan blog.
Add count argument to createMany and createManyQuietly
Jordan Welch contributed the ability to pass an integer to createMany()
and createManyQuietly()
on model factories to create that number of models:
// Before:
$users = User::factory()->createMany([[], [], []]);
// After:
$users = User::factory()->createMany(3);
$this->assertCount(3, $users);
$this->assertInstanceOf(Eloquent\Collection::class, $users);
Release notes
- Add wordWrap to Str in #48012
- Fix assertRedirectToRoute when route uri is empty in #48023
- Fix forced use of write DB connection in #48015
- Add ability to customize class resolution in event discovery in #48031
- Add percentage to Collections in #48034
- Allow Listeners to dynamically specify delay using withDelay in #48026
- Add optional count parameter to createMany and createManyQuietly in #48048
- Add attributes support on default component slot in #48039
- Add WithoutRelations attribute for model serialization in #47989
You can see the complete list of new features and updates below and the diff between 10.18.0 and 10.19.0 on GitHub. The following release notes are directly from the changelog: