Laravel 10.20 Released
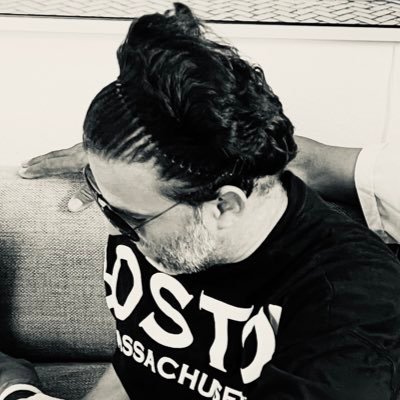
This week, the Laravel team released v10.20 with a createOrFirst() method, benchmark a single callable, a new response JSON assertion, and more:
Eloquent createOrFirst() method
Tony Messias contributed a createOrFirst()
method which attempts to create a new record. If the unique constraint violation occurs, this method attempts to find the matching record:
$result = Model::createOrFirst(
['email' => 'user@example.com'], // Attributes to find
['name' => 'Example User'] // values merged with attributes if created
);
Use trashed relationship even without soft deletes
Mior Muhammad Zaki contributed some updates that support using withTrashed()
, withoutTrashed()
, onlyTrashed()
on a morph relationship:
This would be useful in some 3rd party packages as this has become an issue where it's not possible to define the relationship such as $this->morphTo()->withTrashed() unless all relationship uses SoftDeletes trait...
You can learn more about this change in the description of Pull Request #47880.
Benchmark a single callable and get a result
Tim MacDonald contributed a value()
method to the benchmark utility class, which you can use the measure a given callable inline and get the resulting value:
public function list()
{
[$response, $duration] = Benchmark::value(
fn () => Http::get('https://external-service.com/list.json')
);
if ($duration > 1000) {
Log::warning('external-service.com is running slow');
}
return $response->json();
}
Allow default values when merging data into an API resource
Choraimy Kroonstuiver contributed the ability to pass a default value to mergeWhen()
that will be used. This cleans up logic around mergeWhen()
and mergeUnless
when you want to provide defaults:
Here's an example from the pull request, showing how you'd do this before Laravel 10.20:
class MyResource extends JsonResource
{
public function toArray($request): array
{
return [
/* other properties */
$this->mergeWhen($this->hasSpecificData(), $this->specificData()),
$this->mergeUnless($this->hasSpecificData(), static::genericData()),
];
}
}
And here's what you can do now:
class MyResource extends JsonResource
{
public function toArray($request): array
{
return [
/* other properties */
$this->mergeWhen($this->hasSpecificData(), $this->specificData(), static::genericData()),
];
}
}
Canonical JSON path assertion
Günther Debrauwer contributed a assertJsonPathCanonicalizing()
method you can use to check if a JSON response contains all the expected values without a given order.
$users = User::factory()->count(2)->create();
$response = $this->get('/api/users');
$response->assertJsonPathCanonicalizing(
'data.*.id',
$users->pluck('id')->all()
);
See Pull Request #48117 for more details and example tests that demonstrate this in action.
Release notes
- Allow default values when merging values into a resource in #48073
- Add createOrFirst to Eloquent in #47973
- Allow using *Trashed() methods on MorphTo relationship in #47880
- Add ability to measure a single callable and get result in #48077
- Add assertJsonPathCanonicalizing in #48117
- Configurable storage path via environment variable in #48115
- Support providing subquery as value to where in #48116
You can see the complete list of new features and updates below and the diff between 10.19.0 and 10.20.0 on GitHub. The following release notes are directly from the changelog: