Laravel 10.25 Released
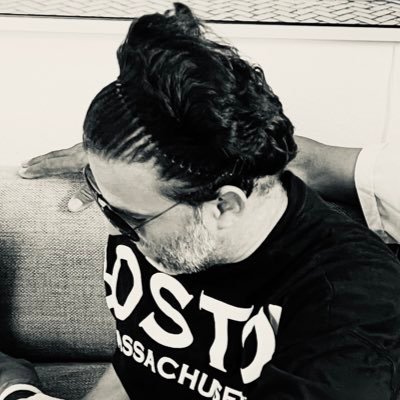
This week, the Laravel team released v10.25 with exception throttling, a Str::take() method, and more. Laravel saw plenty of fixes, updates, and typo fixes this week, which is always appreciated from the community!
Throttle exceptions
Tim MacDonald the ability to throttle exceptions by sampling/rate
limiting exception reporting. Your exception Handler
class can define a throttle()
method which gives you flexibility to rate limit based on an exception type:
// App\Exceptions\Handler
use App\Exceptions\ApiMonitoringException;
use Illuminate\Broadcasting\BroadcastException;
use Illuminate\Cache\RateLimiting\Limit;
use Throwable;
/**
* Throttle incoming exceptions.
*/
protected function throttle(Throwable $e): mixed
{
return match (true) {
$e instanceof BroadcastException => Limit::perMinute(300),
$e instanceof ApiMonitoringException => Lottery::odds(1, 1000),
default => Limit::none(),
};
}
Note that you can return a Limit
which you can use to limit the amount of logs logged (per minute in the example), a Lottery
instance, or simply don't return anything if you want to opt-out for a given exception type.
Check out the documentation on Throttling Reported Exceptions to start using exception throttling in Laravel 10.25.
String take() method
Moshe Brodsky contributed a take()
method to Str
and Stringable
that is syntactic sugar for substr()
when you want to start at the first character:
// Before
str('abcdef')->substr(0, 2) // 'ab'
Str::substr('abcdef', 0, 2); // 'ab'
// After
str('abcdef')->take(2) // 'ab'
Str::take('abcdef', 2); // 'ab'
Increase bcrypt rounds to 12
Stephen Rees-Carter contributed increasing bcrypt
rounds from 10 to 12:
PHP is increasing the default bcrypt cost to either 11 or 12 to keep up with increases in computing, so we should do the same within Laravel. The current default of 10 was set in PHP 11 years ago, which is no longer a suitable default.
12 appears to be the sweet spot between performance and security, as confirmed by a member of the Hashcat team. Symfony uses a cost of 13, however that may be too high for some servers.
See Pull Request #48494 for further details on this change.
Release notes
You can see the complete list of new features and updates below and the diff between 10.24.0 and 10.25.0 on GitHub. The following release notes are directly from the changelog:
- Add take($limit) to Stringables in #48467
- Throttle exceptions in #48391
- Add convertCase to Stringables in #48492
- Increase bcrypt rounds to 12 in #48494
- Set morph type for MorphToMany pivot model in #48432
- Add firstOrCreate and createOrFirst to the HasManyThrough relation in #48541
- Handle custom extensions when caching views in #48524