Assert the Exact JSON Structure of a Response in Laravel 11.19
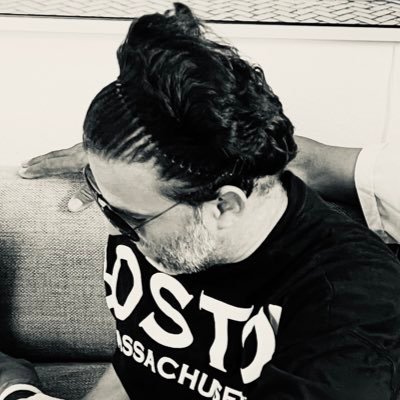
The Laravel team released v11.19 this week, including asserting the exact JSON structure, a whereNone query builder method, a Number::trim() method, HTML assertion convenience methods, and more.
A stand-out contributor in Laravel 11.19 is Günther Debrauwer, who contributed four pull requests to the framework this week!
Add assertExactJsonStructure() Test Method
Günther Debrauwer contributed the assertExactJsonStructure()
to ensure that a test response completely follows an expected structure:
/*
Given the following response:
{
"data": {
"id": 1,
"firstname": "Taylor",
"lastname": "Otwell"
}
}
*/
// This fails
$response->assertExactJsonStructure([
'data' => [
'firstname',
'lastname',
],
]);
// This succeeds
$response->assertExactJsonStructure([
'data' => [
'id',
'firstname',
'lastname',
],
]);
The assertJsonStructure()
method will pass in both of the above examples, so you can use the exact version to ensure the entire shape of the response matches.
Add whereNone Method to the Query Builder
Einar Hansen contributed the whereNone to compliment the whereAny and whereAll methods:
$users = DB::table('users')
->where('active', true)
->whereNone([
'first_name',
'last_name',
'email',
], 'LIKE', 'einar%')
->get();
/*
SELECT *
FROM users
WHERE active = true AND NOT (
first_name LIKE 'einar%' OR
last_name LIKE 'einar%' OR
email LIKE 'einar%'
)
*/
See Pull Request #52260 for more details.
#Add withoutHeader Test Method
Günther Debrauwer contributed a withoutHeader()
method when making test requests. This method could be useful if you have a default header that you want to send with each request but also want to write a test when the header is not present:
$this->withoutHeader('Foo')->get(...);
Add assertSeeHtml Test Methods
Günther Debrauwer contributed three new HTML assertions that are syntactic sugar for assertSee() with escape disabled:
$response->assertSeeHtml('<li>foo</li>');
$response->assertSeeHtmlInOrder([
'<li>foo</li>', '<li>bar</li>', '<li>baz</li>'
]);
$response->assertDontSeeHtml('<li>foo</li>');
// The new methods are equivalent to using `assertSee*` methods without escaping HTML:
$response->assertSee(value: '<li>foo</li>', escape: false);
Add a Number::trim() Method
Günther Debrauwer contributed a Number::trim()
method that trims 0 digits after the decimal point of a given number:
$this->assertSame(12, Number::trim(12));
$this->assertSame(120, Number::trim(120));
$this->assertSame(12, Number::trim(12.0));
$this->assertSame(12.3, Number::trim(12.3));
$this->assertSame(12.3, Number::trim(12.30));
$this->assertSame(12.3456789, Number::trim(12.3456789));
$this->assertSame(12.3456789, Number::trim(12.34567890000));
One use-case in the Pull Request is that json_encode()
will convert a float number that doesn't have any digits after the decimal point:
json_encode(['value' => 4.0, 'another' => 5.21340]);
// = "{"value":4,"another":5.2134}
When asserting these values in a test, you can use the Number::trim()
to match this behavior.
Release notes
You can see the complete list of new features and updates below and the diff between 11.18.0 and 11.19.0 on GitHub. The following release notes are directly from the changelog