Chaperone, Defer, Cache::flexible, and more are now available in Laravel 11.23
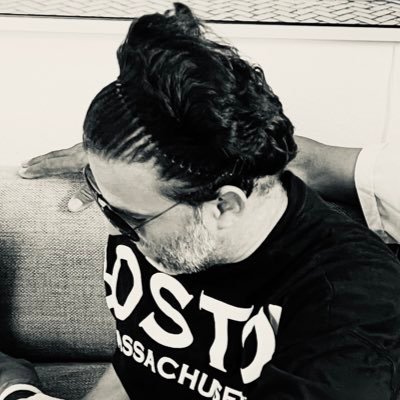
The Laravel team released v11.23 this week, with the Laracon US 2024 open-source updates like defer(), concurrency, contextual container attritubes, and more.
Laracon 2024 Updates
Taylor Otwell contributed all of the goodies he shared in his Laracon US 2024 keynote, including chaperone()
, defer()
, Cache::flexible()
, contextual container attributes, and more.
The documentation is being updated; here are a few highlights you should check out to get familiar with these updates:
- Concurrency Documentation
- Container Contextual Attributes
- Automatically Hydrating Parent Models on Children (AKA
chaperone()
) - Helpers: Deferred Functions
See Pull Request #52710 for full details on everything added related to Taylor's .
Add minRatio and maxRatio Rules to Dimension Validation
Cam Kemshal-Bell added min and max ratio to the dimensions validation rule:
You can use these methods fluently, using minRatio()
, maxRatio()
, and ratioBetween()
:
use Illuminate\Validation\Rule;
//
// Using minRatio and maxRatio fluent methods
//
Rule::dimensions()
->minRatio(1 / 2)
->maxRatio(1 / 3);
// dimensions:min_ratio=0.5,max_ratio=0.33333333333333
//
// Equivalent using ratioBetween()
//
Rule::dimensions()->ratioBetween(min: 1 / 2, max: 1 / 3);
// dimensions:min_ratio=0.5,max_ratio=0.33333333333333
You can also use the string style dimensions rule with this update as well:
use Illuminate\Support\Facades\Validator;
Validator::make($request->all(), [
'min_12' => 'dimensions:min_ratio=1/2',
'square' => 'dimensions:ratio=1',
'max_25' => 'dimensions:max_ratio=2/5',
'min_max' => 'dimensions:min_ratio=1/4,max_ratio=2/5',
]);
Backed Enum Support in Gate Methods and Authorize Middleware
Diaa Fares contributed two pull requests that continue to add backed enum support. This release includes updates to the Gate
methods and Authorize
middleware.
Here are some examples of using Enums with Gate
methods:
enum Abilities: string {
case VIEW_DASHBOARD = 'view-dashboard';
case EDIT = 'edit';
case UPDATE = 'update';
}
// Before
Gate::define('view-dashboard', function (User $user) {
return $user->isAdmin;
});
Gate::authorize('view-dashboard');
Gate::inspect('view-dashboard');
Gate::check('view-dashboard');
Gate::any(['edit', 'update], $post);
Gate::none(['edit', 'update]], $post);
Gate::allows('update', $post);
Gate::denies('update', $post);
// After
Gate::define(Abilities::VIEW_DASHBOARD, function (User $user) {
return $user->isAdmin;
});
Gate::authorize(Abilities::VIEW_DASHBOARD);
Gate::inspect(Abilities::VIEW_DASHBOARD);
Gate::check(Abilities::VIEW_DASHBOARD);
Gate::any([Abilities::EDIT, Abilities::UPDATE], $post);
Gate::none([Abilities::EDIT, Abilities::UPDATE], $post);
Gate::allows(Abilities::UPDATE, $post);
Gate::denies(Abilities::UPDATE, $post);
Here's an example of the Authorize
middleware's support for backed enums:
Route::get('/dashboard', [AdminDashboardController::class, 'index'])
->middleware(
Authorize::using(Abilities::VIEW_DASHBOARD)
)
->name(AdminRoutes::DASHBOARD);
Skip Middleware for Queue Jobs
Kennedy Tedesco contributed a Skip middleware to skip a job based on a condition. This middleware has three static constructor methods you can use, including when()
, unless()
. The job is skipped based on the result of the condition used:
class MyJob implements ShouldQueue
{
use Queueable;
public function handle(): void
{
// TODO
}
public function middleware(): array
{
return [
Skip::when($someCondition), // Skip when `true`
Skip::unless($someCondition), // Skip when `false`
Skip::when(function(): bool {
if ($someCondition) {
return true;
}
return false;
}),
];
}
}
Eloquent Collection findOrFail() Method
Steve Bauman contributed a findOrFail()
method on Eloquent collections that adds a way to find a model on an already populated collection:
$users = User::get(); // [User(id: 1), User(id: 2)]
$users->findOrFail(1); // User
$user->findOrFail([]); // []
$user->findOrFail([1, 2]); // [User, User]
$user->findOrFail(3); // ModelNotFoundException: 'No query results for model [User] 3'
$user->findOrFail([1, 2, 3]); // ModelNotFoundException: 'No query results for model [User] 3'
Release notes
You can see the complete list of new features and updates below and the diff between 11.22.0 and 11.23.0 on GitHub. The following release notes are directly from the changelog: