Laravel 8.77 Just Released
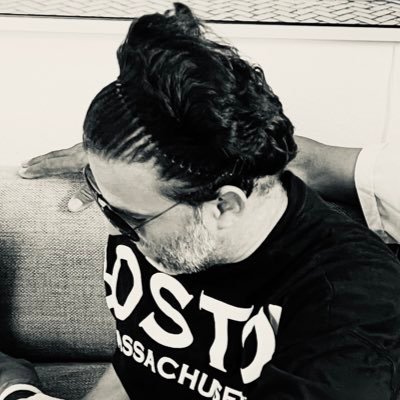
The Laravel team released 8.77 with attribute cast/accessor improvements, a request date() method to access data as a DateTime instance, MAC address validation, the ability to define custom temporary URLs on storage disks, and the latest changes in the v8.x branch.
Attribute Cast / Accessor Improvements
Taylor Otwell contributed a new way to define attribute accessors and mutators:
// Old, two-method approach
public function setTitleAttribute($value)
{
$this->attributes['title'] = strtolower($value);
}
// New Approach
protected function title(): Attribute
{
return new Attribute(
set: fn ($value) => strtolower($value),
);
}
Here's an example with both a get and set implementation:
/**
* Get the user's title.
*/
protected function title(): Attribute
{
return new Attribute(
get: fn ($value) => strtoupper($value),
set: fn ($value) => strtolower($value),
);
}
To learn more, check out the pull request description and discussion around this feature.
DateTime Parsing Added to the Request Instance
@Italo contributed a date() method to the request instance, making it convenient to get a date instance from request data:
// Before
if ($date = $request->input('when')) {
$date = Carbon::parse($datetime);
}
// After
$date = $request->date('when');
Per-connection Predis Prefixes
Ben Tidy contributed the ability to use prefixes on a per-connection basis for Predis. Here’s an example from the pull request of Redis configuration:
'redis' => [
'client' => env('REDIS_CLIENT', 'predis'),
'default' => [
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => env('REDIS_DB', 0),
'prefix' => env('REDIS_PREFIX', 'prefix:'),
],
'cache' => [
'host' => env('REDIS_HOST', '127.0.0.1'),
'password' => env('REDIS_PASSWORD', null),
'port' => env('REDIS_PORT', 6379),
'database' => env('REDIS_CACHE_DB', 1),
'prefix' => env('REDIS_PREFIX', 'prefix2:'),
],
],
MAC Address Validation Rule
Bilal Al-Massry contributed a mac_address validation rule, to validate a MAC address:
$trans = $this->getIlluminateArrayTranslator();
$v = new Validator($trans, ['mac' => 'foo'], ['mac' => 'mac_address']);
$this->assertFalse($v->passes());
$trans = $this->getIlluminateArrayTranslator();
$v = new Validator($trans, ['mac' => '01-23-45-67-89-ab'], ['mac' => 'mac_address']);
$this->assertTrue($v->passes());
$trans = $this->getIlluminateArrayTranslator();
$v = new Validator($trans, ['mac' => '01-23-45-67-89-AB'], ['mac' => 'mac_address']);
$this->assertTrue($v->passes());
Define Temporary URL Method for Storage
Ash Allen contributed to the ability to define a custom temporary URL logic for the Storage facade. Here are a few examples from the pull request:
Storage::disk('local')
->buildTemporaryUrlUsing(function ($path, $expiration, $options) {
return 'using local';
});
// $url is: 'using local'
$url = Storage::temporaryUrl('file.jpg', now()->addMinutes(5));
Release Notes
You can see the complete list of new features and updates below and the diff between 8.76.0 and 8.77.0 on GitHub. The following release notes are directly from the changelog:
v8.77.0
Added
- Attribute Cast / Accessor Improvements (#40022)
- Added Illuminate/View/Factory::renderUnless() (#40077)
- Added datetime parsing to Request instance (#39945)
- Make it possible to use prefixes on Predis per Connection (#40083)
- Added rule to validate MAC address (#40098)
- Added ability to define temporary URL macro for storage (#40100)
Fixed
- Fixed possible out of memory error when deleting values by reference key from cache in Redis driver (#40039)
- Added Illuminate/Filesystem/FilesystemManager::setApplication() (#40058)
- Fixed arg passing in doesntContain (739d847)
- Translate Enum rule message (#40089)
- Fixed date validation (#40088)
- Don’t allow models and except together in PruneCommand.php (f62fe66)
Changed
- Passthru Eloquent\Query::explain function to Query\Builder:explain for the ability to use database-specific explain commands (#40075)