Laravel 9.16 Released
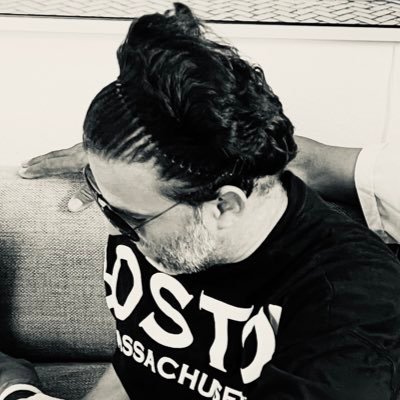
The Laravel team released v9.16 with additional UUID testing helpers, a new Eloquent method withWhereHas(), user authentication for Pusher, and more:
UUID testing helpers
Tim MacDonald contributed additional UUID testing helpers to the generation of UUIDs via the Str helper. Currently, you might capture a UUID first or fake a specific UUID like so:
$uuid = Str::uuid();
Str::createUuidsUsing(fn () => $uuid);
Str::uuid() === Str::uuid() === $uuid;
// Cleanup
Str::createUuidsNormally();
With this PR now, you can do the following:
Str::freezeUuids();
// do stuff...
Str::uuid() === Str::uuid() == $uuid;
// cleanup...
Str:: createUuidsNormally();
You can also pass a closure to only freeze creation for the duration of the closure:
Str::freezeUuids(function ($uuid) {
// do stuff...
Str::uuid() === Str::uuid() === $uuid;
});
You can also return a sequence of UUIDs:
Str::createUuidsUsingSequence([
$zeroth = Str::uuid(),
$first = Str::uuid(),
]);
Str::uuid() === $zeroth;
Str::uuid() === $first;
Str::uuid(); // back to random UUIDs
If you'd like to learn more and see the implementation details, check out Pull Request #42619.
Eloquent withWhereHas()
@eusonlito contributed a withWhereHas() method that simplifies cases where you have to repeat code used to both filter with whereHas and select the same record via with():
CollectionModel::whereHas('products', function ($query) {
$query->where('enabled', true)->where('sale', true);
})->with(['products' => function ($query) {
$query->where('enabled', true)->where('sale', true);
});
Using the withWhereHas
method, you can simplify your code around this use case:
CollectionModel::withWhereHas('products', fn ($query) => $query->where('enabled', true)->where('sale', true));
User authentication for Pusher
@rennokki contributed user authentication for Pusher:
Pusher recently launched a feature that allows their users to enable authentication for connections, alongside the channel authorizations: https://pusher.com/docs/channels/using_channels/user-authentication/
Shortly, it's a feature that will make sure that anyone connecting to the websockets should be an authenticated user within the app. This will increase the trust of your connected users and you can broadcast events to user's connections by their database ID, instead of the usual Socket ID.
See Pull Request #42531 for further details on discussion and implementation around this feature. Also, a documentation pull request is open at the time of publication which has more information on how to use this feature.
Release Notes
You can see the complete list of new features and updates below and the diff between 9.15.0 and 9.16.0 on GitHub. The following release notes are directly from the changelog
Added
- Added Eloquent withWhereHas method (#42597)
- User authentication for Pusher (#42531)
- Added additional uuid testing helpers (#42619)
Fixed
- Fix queueable notification's ID overwritten (#42581)
- Handle undefined array key error in route (#42606)
Deprecated
- Illuminate/Routing/Redirector::home() (#42600)