Why using Laravel Eloquent?
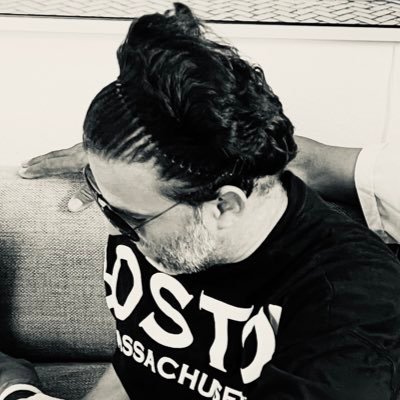
Eloquent is used in Laravel for several key reasons that contribute to its popularity and effectiveness in application development.
Eloquent is the ORM (Object-Relational Mapping) tool used in Laravel for several compelling reasons:
Simplicity and Readability
Eloquent uses a straightforward and human-readable syntax that is clean and expressive, making it easy to write and understand database interactions. For example, retrieving all users can be done with a simple line of code:
$users = User::all();
Eloquent employs an object-oriented approach that aligns seamlessly with PHP and modern development practices. This allows you to interact with database records as if they were regular PHP objects, making the code more intuitive and easier to understand.
$user = User::find(1);
echo $user->name;
Active Record Pattern
Eloquent follows the Active Record design pattern, where each model represents a database table and each instance of the model corresponds to a single row in that table. This makes it intuitive to work with database records as objects and simplifies CRUD (Create, Read, Update, Delete) operations, allowing developers to perform these tasks easily.
$user = new User;
$user->name = 'John Doe';
$user->save();
Relationship Management
Eloquent makes it easy to define and work with relationships between different models, such as one-to-one, one-to-many, and many-to-many relationships. This simplifies complex database interactions.
// Define a relationship in the User model
public function posts()
{
return $this->hasMany(Post::class);
}
// Accessing related data
$user = User::find(1);
$posts = $user->posts;
Built-in Methods
Eloquent provides a wide range of built-in methods for common database operations, such as querying, inserting, updating, and deleting records. This reduces the need to write repetitive code.
$user = User::findOrFail(1);
$user->delete();
Eager Loading
Eloquent supports eager loading, which helps optimize database queries by loading related models in a single query. This can improve performance by reducing the number of queries executed.
Mutators and Accessors
Eloquent allows developers to define mutators and accessors, enabling automatic manipulation of model attributes when they are retrieved from or stored in the database.
// Defining an accessor in the User model
public function getFullNameAttribute()
{
return "{$this->first_name} {$this->last_name}";
}
// Using the accessor
$user = User::find(1);
echo $user->full_name;
Scopes
Eloquent supports query scopes, which allow for reusable chunks of query logic. This promotes code reuse and helps keep queries clean and maintainable.
// Defining a scope in the User model
public function scopeActive($query)
{
return $query->where('status', 'active');
}
// Using the scope
$activeUsers = User::active()->get();
Timestamps and Soft Deletes
Eloquent includes built-in support for timestamps (created_at and updated_at) and soft deletes (deleted_at), simplifying the management of these common fields.
// Soft deleting a post
$post = Post::find(1);
$post->delete(); // The post is not removed from the database, only marked as deleted
// Retrieving only non-deleted posts
$posts = Post::all(); // This will not include soft deleted posts
// Retrieving soft deleted posts
$deletedPosts = Post::onlyTrashed()->get();
// Restoring a soft deleted post
$deletedPost = Post::withTrashed()->find(1);
$deletedPost->restore(); // The post is now restored and will be included in the default query results
// Permanently deleting a post
$deletedPost->forceDelete(); // The post is permanently removed from the database
Integration with Laravel Ecosystem
Eloquent integrates smoothly with other components of the Laravel ecosystem, including validation, form requests, and resource controllers, ensuring a cohesive and consistent development experience.
Overall, Eloquent offers a powerful and flexible approach for developers to interact with databases, making it a popular choice for many Laravel applications. However, it is true that using Eloquent in Laravel can introduce some performance overhead compared to writing raw SQL or using Laravel's Query Builder. The impact on performance is typically minimal and largely depends on how Eloquent is utilized. Here are some factors to consider:
Abstraction Overhead
Eloquent adds an abstraction layer that makes database interaction easier but introduces some overhead compared to raw SQL.
Lazy Loading vs. Eager Loading
By default, Eloquent uses lazy loading, which can result in multiple queries when accessing related models. Using eager loading (the with
method) can mitigate this by retrieving related models in fewer queries.
Complex Queries
For very complex queries, raw SQL or Query Builder can be more efficient. Eloquent's simplicity can sometimes lead to suboptimal queries for complex scenarios.
Query Optimization
Regardless of the method used, proper indexing, query optimization, and caching strategies are crucial for performance. And here is where Eloquent plays an important role.
N+1 Problem
Eloquent can easily lead to issues related to normal forms if relationships are not managed properly. Eager loading can help reduce this problem.
Although Eloquent may introduce some performance overhead, it offers significant benefits in terms of readability, maintainability, and rapid development. The performance trade-off is often worth it for many applications, especially when proper optimization techniques are applied.