PHP 8.4 released
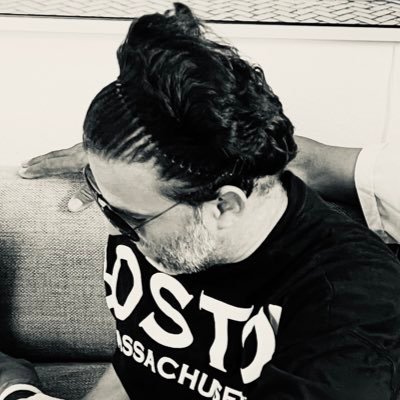
The release of PHP 8.4 brings exciting new features, enhancements, and performance improvements that promise to make PHP development faster, cleaner, and more efficient. In this article, we'll explore some of the standout features of PHP 8.4, their benefits, and examples to showcase how they work.
Key Features in PHP 8.4
1. Readonly Properties 2.0
PHP 8.4 expands the capabilities of readonly properties introduced in PHP 8.1. Now, readonly properties support initialization via constructors even when extending base classes, streamlining inheritance.
Example:
class BaseClass {
public readonly string $baseProperty;
public function __construct(string $value) {
$this->baseProperty = $value;
}
}
class ChildClass extends BaseClass {
public readonly string $childProperty;
public function __construct(string $baseValue, string $childValue) {
parent::__construct($baseValue);
$this->childProperty = $childValue;
}
}
$instance = new ChildClass("BaseValue", "ChildValue");
echo $instance->baseProperty; // Output: BaseValue
echo $instance->childProperty; // Output: ChildValue
2. Conditional Break in foreach
Loops
PHP 8.4 introduces a break if
statement that allows conditional termination of a loop without requiring an additional if
block, making code cleaner and more readable.
Example:
$numbers = [1, 2, 3, 4, 5];
foreach ($numbers as $number) {
echo $number . PHP_EOL;
break if ($number === 3); // Loop terminates when $number equals 3
}
3. New Standalone String Functions
PHP 8.4 introduces new string manipulation functions to simplify common tasks, such as str_insert
.
Example:
$string = "Hello World!";
$updatedString = str_insert($string, " Beautiful", 5); // Insert " Beautiful" at position 5
echo $updatedString; // Output: Hello Beautiful World!
4. Simplified JSON Handling
A new json_validate
function is introduced to quickly verify if a string is valid JSON, improving error handling in JSON operations.
Example:
$jsonString = '{"key": "value"}';
if (json_validate($jsonString)) {
echo "Valid JSON!";
} else {
echo "Invalid JSON!";
}
5. Enhanced Attributes for Annotations
Attributes are now more expressive with support for variadic arguments and conditional application. This enhances the meta-programming capabilities of PHP.
Example:
#[MyAttribute('value1', 'value2', condition: true)]
class MyClass {}
6. Performance Improvements
PHP 8.4 includes significant optimizations for memory usage and execution time. Functions like array_map
and array_filter
are now more performant, further speeding up PHP applications.
Why Upgrade to PHP 8.4?
- Cleaner Syntax: Features like
break if
and improved readonly properties make your code easier to read and maintain. - Improved Developer Experience: Tools like
str_insert
andjson_validate
reduce boilerplate code. - Better Performance: Faster execution ensures your applications are more responsive.
- Future-Proofing: Staying current with PHP ensures compatibility with the latest libraries and tools.
Conclusion
PHP 8.4 builds on the foundation of earlier versions with thoughtful additions that enhance productivity, readability, and performance. Whether you're working on legacy systems or greenfield projects, the features in PHP 8.4 are worth exploring.
Make sure to test your applications thoroughly before upgrading, as some features might require adjustments for backward compatibility.